The documentation you are viewing is for Dapr v1.8 which is an older version of Dapr. For up-to-date documentation, see the latest version.
Quickstart: Service Invocation
With Dapr’s Service Invocation building block, your application can communicate reliably and securely with other applications.
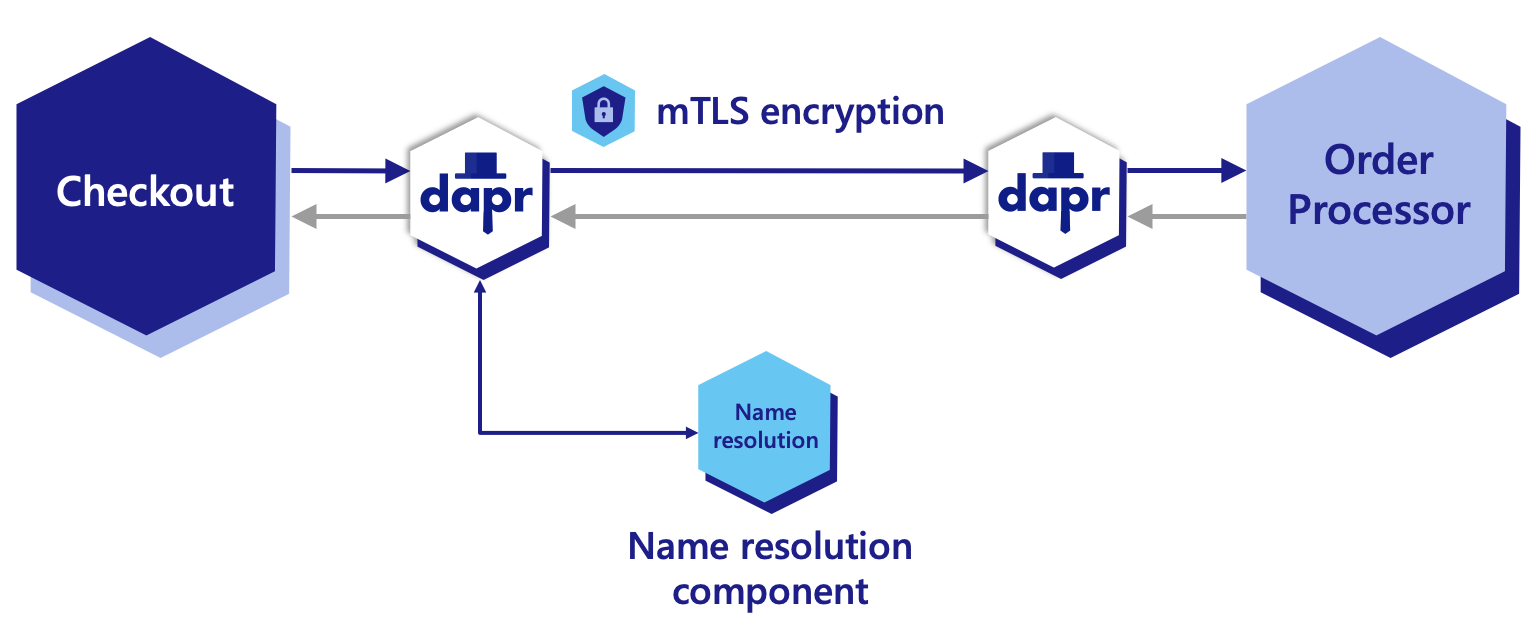
Dapr offers several methods for service invocation, which you can choose depending on your scenario. For this Quickstart, you’ll enable the checkout service to invoke a method using HTTP proxy in the order-processor service.
Learn more about Dapr’s methods for service invocation in the overview article.
Select your preferred language before proceeding with the Quickstart.
Step 1: Pre-requisites
For this example, you will need:
Step 2: Set up the environment
Clone the sample provided in the Quickstarts repo.
git clone https://github.com/dapr/quickstarts.git
Step 3: Run order-processor
service
In a terminal window, from the root of the Quickstart clone directory
navigate to order-processor
directory.
cd service_invocation/python/http/order-processor
Install the dependencies and build the application:
pip3 install -r requirements.txt
Run the order-processor
service alongside a Dapr sidecar.
dapr run --app-port 8001 --app-id order-processor --app-protocol http --dapr-http-port 3501 -- python3 app.py
Note: Since Python3.exe is not defined in Windows, you may need to use
python app.py
instead ofpython3 app.py
.
@app.route('/orders', methods=['POST'])
def getOrder():
data = request.json
print('Order received : ' + json.dumps(data), flush=True)
return json.dumps({'success': True}), 200, {
'ContentType': 'application/json'}
app.run(port=8001)
Step 4: Run checkout
service
In a new terminal window, from the root of the Quickstart clone directory
navigate to the checkout
directory.
cd service_invocation/python/http/checkout
Install the dependencies and build the application:
pip3 install -r requirements.txt
Run the checkout
service alongside a Dapr sidecar.
dapr run --app-id checkout --app-protocol http --dapr-http-port 3500 -- python3 app.py
Note: Since Python3.exe is not defined in Windows, you may need to use
python app.py
instead ofpython3 app.py
.
In the checkout
service, you’ll notice there’s no need to rewrite your app code to use Dapr’s service invocation. You can enable service invocation by simply adding the dapr-app-id
header, which specifies the ID of the target service.
headers = {'dapr-app-id': 'order-processor'}
result = requests.post(
url='%s/orders' % (base_url),
data=json.dumps(order),
headers=headers
)
Step 5: View the Service Invocation outputs
Dapr invokes an application on any Dapr instance. In the code, the sidecar programming model encourages each application to talk to its own instance of Dapr. The Dapr instances then discover and communicate with one another.
checkout
service output:
== APP == Order passed: {"orderId": 1}
== APP == Order passed: {"orderId": 2}
== APP == Order passed: {"orderId": 3}
== APP == Order passed: {"orderId": 4}
== APP == Order passed: {"orderId": 5}
== APP == Order passed: {"orderId": 6}
== APP == Order passed: {"orderId": 7}
== APP == Order passed: {"orderId": 8}
== APP == Order passed: {"orderId": 9}
== APP == Order passed: {"orderId": 10}
order-processor
service output:
== APP == Order received: {"orderId": 1}
== APP == Order received: {"orderId": 2}
== APP == Order received: {"orderId": 3}
== APP == Order received: {"orderId": 4}
== APP == Order received: {"orderId": 5}
== APP == Order received: {"orderId": 6}
== APP == Order received: {"orderId": 7}
== APP == Order received: {"orderId": 8}
== APP == Order received: {"orderId": 9}
== APP == Order received: {"orderId": 10}
Step 1: Pre-requisites
For this example, you will need:
Step 2: Set up the environment
Clone the sample provided in the Quickstarts repo.
git clone https://github.com/dapr/quickstarts.git
Step 3: Run order-processor
service
In a terminal window, from the root of the Quickstart clone directory
navigate to order-processor
directory.
cd service_invocation/javascript/http/order-processor
Install the dependencies:
npm install
Run the order-processor
service alongside a Dapr sidecar.
dapr run --app-port 5001 --app-id order-processor --app-protocol http --dapr-http-port 3501 -- npm start
app.post('/orders', (req, res) => {
console.log("Order received:", req.body);
res.sendStatus(200);
});
Step 4: Run checkout
service
In a new terminal window, from the root of the Quickstart clone directory
navigate to the checkout
directory.
cd service_invocation/javascript/http/checkout
Install the dependencies:
npm install
Run the checkout
service alongside a Dapr sidecar.
dapr run --app-id checkout --app-protocol http --dapr-http-port 3500 -- npm start
In the checkout
service, you’ll notice there’s no need to rewrite your app code to use Dapr’s service invocation. You can enable service invocation by simply adding the dapr-app-id
header, which specifies the ID of the target service.
let axiosConfig = {
headers: {
"dapr-app-id": "order-processor"
}
};
const res = await axios.post(`${DAPR_HOST}:${DAPR_HTTP_PORT}/orders`, order , axiosConfig);
console.log("Order passed: " + res.config.data);
Step 5: View the Service Invocation outputs
Dapr invokes an application on any Dapr instance. In the code, the sidecar programming model encourages each application to talk to its own instance of Dapr. The Dapr instances then discover and communicate with one another.
checkout
service output:
== APP == Order passed: {"orderId": 1}
== APP == Order passed: {"orderId": 2}
== APP == Order passed: {"orderId": 3}
== APP == Order passed: {"orderId": 4}
== APP == Order passed: {"orderId": 5}
== APP == Order passed: {"orderId": 6}
== APP == Order passed: {"orderId": 7}
== APP == Order passed: {"orderId": 8}
== APP == Order passed: {"orderId": 9}
== APP == Order passed: {"orderId": 10}
order-processor
service output:
== APP == Order received: {"orderId": 1}
== APP == Order received: {"orderId": 2}
== APP == Order received: {"orderId": 3}
== APP == Order received: {"orderId": 4}
== APP == Order received: {"orderId": 5}
== APP == Order received: {"orderId": 6}
== APP == Order received: {"orderId": 7}
== APP == Order received: {"orderId": 8}
== APP == Order received: {"orderId": 9}
== APP == Order received: {"orderId": 10}
Step 1: Pre-requisites
For this example, you will need:
Step 2: Set up the environment
Clone the sample provided in the Quickstarts repo.
git clone https://github.com/dapr/quickstarts.git
Step 3: Run order-processor
service
In a terminal window, from the root of the Quickstart clone directory
navigate to order-processor
directory.
cd service_invocation/csharp/http/order-processor
Install the dependencies:
dotnet restore
dotnet build
Run the order-processor
service alongside a Dapr sidecar.
dapr run --app-port 7001 --app-id order-processor --app-protocol http --dapr-http-port 3501 -- dotnet run
app.MapPost("/orders", async context => {
var data = await context.Request.ReadFromJsonAsync<Order>();
Console.WriteLine("Order received : " + data);
await context.Response.WriteAsync(data.ToString());
});
Step 4: Run checkout
service
In a new terminal window, from the root of the Quickstart clone directory
navigate to the checkout
directory.
cd service_invocation/csharp/http/checkout
Install the dependencies:
dotnet restore
dotnet build
Run the checkout
service alongside a Dapr sidecar.
dapr run --app-id checkout --app-protocol http --dapr-http-port 3500 -- dotnet run
In the checkout
service, you’ll notice there’s no need to rewrite your app code to use Dapr’s service invocation. You can enable service invocation by simply adding the dapr-app-id
header, which specifies the ID of the target service.
var client = new HttpClient();
client.DefaultRequestHeaders.Accept.Add(new System.Net.Http.Headers.MediaTypeWithQualityHeaderValue("application/json"));
client.DefaultRequestHeaders.Add("dapr-app-id", "order-processor");
var response = await client.PostAsync($"{baseURL}/orders", content);
Console.WriteLine("Order passed: " + order);
Step 5: View the Service Invocation outputs
Dapr invokes an application on any Dapr instance. In the code, the sidecar programming model encourages each application to talk to its own instance of Dapr. The Dapr instances then discover and communicate with one another.
checkout
service output:
== APP == Order passed: Order { OrderId: 1 }
== APP == Order passed: Order { OrderId: 2 }
== APP == Order passed: Order { OrderId: 3 }
== APP == Order passed: Order { OrderId: 4 }
== APP == Order passed: Order { OrderId: 5 }
== APP == Order passed: Order { OrderId: 6 }
== APP == Order passed: Order { OrderId: 7 }
== APP == Order passed: Order { OrderId: 8 }
== APP == Order passed: Order { OrderId: 9 }
== APP == Order passed: Order { OrderId: 10 }
order-processor
service output:
== APP == Order received: Order { OrderId: 1 }
== APP == Order received: Order { OrderId: 2 }
== APP == Order received: Order { OrderId: 3 }
== APP == Order received: Order { OrderId: 4 }
== APP == Order received: Order { OrderId: 5 }
== APP == Order received: Order { OrderId: 6 }
== APP == Order received: Order { OrderId: 7 }
== APP == Order received: Order { OrderId: 8 }
== APP == Order received: Order { OrderId: 9 }
== APP == Order received: Order { OrderId: 10 }
Step 1: Pre-requisites
For this example, you will need:
- Dapr CLI and initialized environment.
- Java JDK 11 (or greater):
- Oracle JDK, or
- OpenJDK
- Apache Maven, version 3.x.
Step 2: Set up the environment
Clone the sample provided in the Quickstarts repo.
git clone https://github.com/dapr/quickstarts.git
Step 3: Run order-processor
service
In a terminal window, from the root of the Quickstart clone directory
navigate to order-processor
directory.
cd service_invocation/java/http/order-processor
Install the dependencies:
mvn clean install
Run the order-processor
service alongside a Dapr sidecar.
dapr run --app-id order-processor --app-port 9001 --app-protocol http --dapr-http-port 3501 -- java -jar target/OrderProcessingService-0.0.1-SNAPSHOT.jar
public String processOrders(@RequestBody Order body) {
System.out.println("Order received: "+ body.getOrderId());
return "CID" + body.getOrderId();
}
Step 4: Run checkout
service
In a new terminal window, from the root of the Quickstart clone directory
navigate to the checkout
directory.
cd service_invocation/java/http/checkout
Install the dependencies:
mvn clean install
Run the checkout
service alongside a Dapr sidecar.
dapr run --app-id checkout --app-protocol http --dapr-http-port 3500 -- java -jar target/CheckoutService-0.0.1-SNAPSHOT.jar
In the checkout
service, you’ll notice there’s no need to rewrite your app code to use Dapr’s service invocation. You can enable service invocation by simply adding the dapr-app-id
header, which specifies the ID of the target service.
.header("Content-Type", "application/json")
.header("dapr-app-id", "order-processor")
HttpResponse<String> response = httpClient.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println("Order passed: "+ orderId)
Step 5: View the Service Invocation outputs
Dapr invokes an application on any Dapr instance. In the code, the sidecar programming model encourages each application to talk to its own instance of Dapr. The Dapr instances then discover and communicate with one another.
checkout
service output:
== APP == Order passed: 1
== APP == Order passed: 2
== APP == Order passed: 3
== APP == Order passed: 4
== APP == Order passed: 5
== APP == Order passed: 6
== APP == Order passed: 7
== APP == Order passed: 8
== APP == Order passed: 9
== APP == Order passed: 10
order-processor
service output:
== APP == Order received: 1
== APP == Order received: 2
== APP == Order received: 3
== APP == Order received: 4
== APP == Order received: 5
== APP == Order received: 6
== APP == Order received: 7
== APP == Order received: 8
== APP == Order received: 9
== APP == Order received: 10
Step 1: Pre-requisites
For this example, you will need:
Step 2: Set up the environment
Clone the sample provided in the Quickstarts repo.
git clone https://github.com/dapr/quickstarts.git
Step 3: Run order-processor
service
In a terminal window, from the root of the Quickstart clone directory
navigate to order-processor
directory.
cd service_invocation/go/http/order-processor
Install the dependencies:
go build .
Run the order-processor
service alongside a Dapr sidecar.
dapr run --app-port 6001 --app-id order-processor --app-protocol http --dapr-http-port 3501 -- go run .
Each order is received via an HTTP POST request and processed by the
getOrder
function.
func getOrder(w http.ResponseWriter, r *http.Request) {
data, err := ioutil.ReadAll(r.Body)
if err != nil {
log.Fatal(err)
}
log.Printf("Order received : %s", string(data))
}
Step 4: Run checkout
service
In a new terminal window, from the root of the Quickstart clone directory
navigate to the checkout
directory.
cd service_invocation/go/http/checkout
Install the dependencies:
go build .
Run the checkout
service alongside a Dapr sidecar.
dapr run --app-id checkout --app-protocol http --dapr-http-port 3500 -- go run .
In the checkout
service, you’ll notice there’s no need to rewrite your app code to use Dapr’s service invocation. You can enable service invocation by simply adding the dapr-app-id
header, which specifies the ID of the target service.
req.Header.Add("dapr-app-id", "order-processor")
response, err := client.Do(req)
Step 5: View the Service Invocation outputs
Dapr invokes an application on any Dapr instance. In the code, the sidecar programming model encourages each application to talk to its own instance of Dapr. The Dapr instances then discover and communicate with one another.
checkout
service output:
== APP == Order passed: {"orderId":1}
== APP == Order passed: {"orderId":2}
== APP == Order passed: {"orderId":3}
== APP == Order passed: {"orderId":4}
== APP == Order passed: {"orderId":5}
== APP == Order passed: {"orderId":6}
== APP == Order passed: {"orderId":7}
== APP == Order passed: {"orderId":8}
== APP == Order passed: {"orderId":9}
== APP == Order passed: {"orderId":10}
order-processor
service output:
== APP == Order received : {"orderId":1}
== APP == Order received : {"orderId":2}
== APP == Order received : {"orderId":3}
== APP == Order received : {"orderId":4}
== APP == Order received : {"orderId":5}
== APP == Order received : {"orderId":6}
== APP == Order received : {"orderId":7}
== APP == Order received : {"orderId":8}
== APP == Order received : {"orderId":9}
== APP == Order received : {"orderId":10}
Tell us what you think!
We’re continuously working to improve our Quickstart examples and value your feedback. Did you find this Quickstart helpful? Do you have suggestions for improvement?
Join the discussion in our discord channel.
Next Steps
- Learn more about Service Invocation as a Dapr building block
- Learn more about how to invoke Dapr’s Service Invocation with:
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.