The documentation you are viewing is for Dapr v1.8 which is an older version of Dapr. For up-to-date documentation, see the latest version.
Quickstart: Secrets Management
Dapr provides a dedicated secrets API that allows developers to retrieve secrets from a secrets store. In this quickstart, you:
- Run a microservice with a secret store component.
- Retrieve secrets using the Dapr secrets API in the application code.
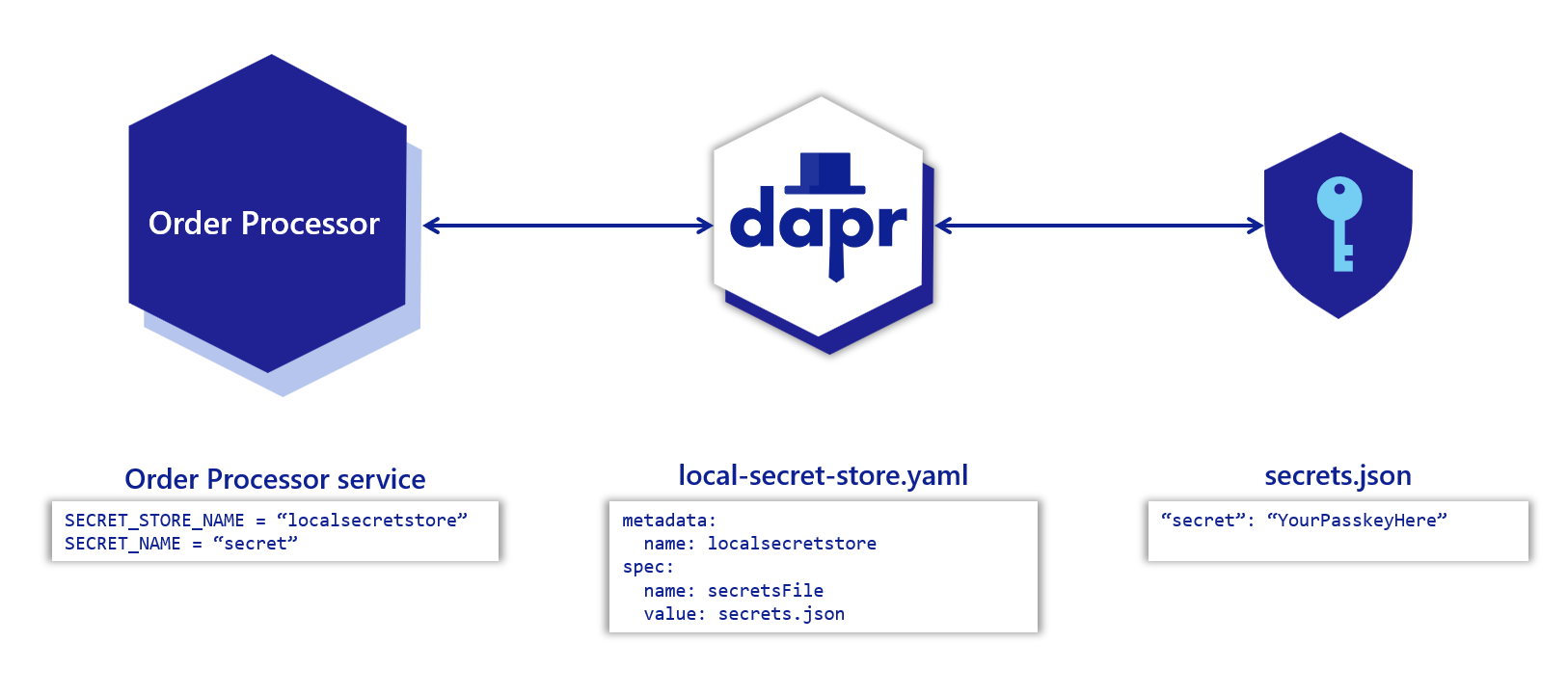
Select your preferred language-specific Dapr SDK before proceeding with the Quickstart.
Pre-requisites
For this example, you will need:
Step 1: Set up the environment
Clone the sample provided in the Quickstarts repo.
git clone https://github.com/dapr/quickstarts.git
Step 2: Retrieve the secret
In a terminal window, navigate to the order-processor
directory.
cd secrets_management/python/sdk/order-processor
Install the dependencies:
pip3 install -r requirements.txt
Run the order-processor
service alongside a Dapr sidecar.
dapr run --app-id order-processor --components-path ../../../components/ -- python3 app.py
Note: Since Python3.exe is not defined in Windows, you may need to use
python app.py
instead ofpython3 app.py
.
Behind the scenes
order-processor
service
Notice how the order-processor
service below points to:
- The
DAPR_SECRET_STORE
defined in thelocal-secret-store.yaml
component. - The secret defined in
secrets.json
.
# app.py
DAPR_SECRET_STORE = 'localsecretstore'
SECRET_NAME = 'secret'
with DaprClient() as client:
secret = client.get_secret(store_name=DAPR_SECRET_STORE, key=SECRET_NAME)
logging.info('Fetched Secret: %s', secret.secret)
local-secret-store.yaml
component
DAPR_SECRET_STORE
is defined in the local-secret-store.yaml
component file, located in secrets_management/components:
apiVersion: dapr.io/v1alpha1
kind: Component
metadata:
name: localsecretstore
namespace: default
spec:
type: secretstores.local.file
version: v1
metadata:
- name: secretsFile
value: secrets.json
- name: nestedSeparator
value: ":"
In the YAML file:
metadata/name
is how your application references the component (calledDAPR_SECRET_STORE
in the code sample).spec/metadata
defines the connection to the secret used by the component.
secrets.json
file
SECRET_NAME
is defined in the secrets.json
file, located in secrets_management/python/sdk/order-processor:
{
"secret": "YourPasskeyHere"
}
Step 3: View the order-processor outputs
As specified in the application code above, the order-processor
service retrieves the secret via the Dapr secret store and displays it in the console.
Order-processor output:
== APP == INFO:root:Fetched Secret: {'secret': 'YourPasskeyHere'}
Pre-requisites
For this example, you will need:
Step 1: Set up the environment
Clone the sample provided in the Quickstarts repo.
git clone https://github.com/dapr/quickstarts.git
Step 2: Retrieve the secret
In a terminal window, navigate to the order-processor
directory.
cd secrets_management/javascript/sdk/order-processor
Install the dependencies:
npm install
Run the order-processor
service alongside a Dapr sidecar.
dapr run --app-id order-processor --components-path ../../../components/ -- npm start
Behind the scenes
order-processor
service
Notice how the order-processor
service below points to:
- The
DAPR_SECRET_STORE
defined in thelocal-secret-store.yaml
component. - The secret defined in
secrets.json
.
// index.js
const DAPR_SECRET_STORE = "localsecretstore";
const SECRET_NAME = "secret";
async function main() {
// ...
const secret = await client.secret.get(DAPR_SECRET_STORE, SECRET_NAME);
console.log("Fetched Secret: " + JSON.stringify(secret));
}
local-secret-store.yaml
component
DAPR_SECRET_STORE
is defined in the local-secret-store.yaml
component file, located in secrets_management/components:
apiVersion: dapr.io/v1alpha1
kind: Component
metadata:
name: localsecretstore
namespace: default
spec:
type: secretstores.local.file
version: v1
metadata:
- name: secretsFile
value: secrets.json
- name: nestedSeparator
value: ":"
In the YAML file:
metadata/name
is how your application references the component (calledDAPR_SECRET_STORE
in the code sample).spec/metadata
defines the connection to the secret used by the component.
secrets.json
file
SECRET_NAME
is defined in the secrets.json
file, located in secrets_management/javascript/sdk/order-processor:
{
"secret": "YourPasskeyHere"
}
Step 3: View the order-processor outputs
As specified in the application code above, the order-processor
service retrieves the secret via the Dapr secret store and displays it in the console.
Order-processor output:
== APP ==
== APP == > order-processor@1.0.0 start
== APP == > node index.js
== APP ==
== APP == Fetched Secret: {"secret":"YourPasskeyHere"}
Pre-requisites
For this example, you will need:
Step 1: Set up the environment
Clone the sample provided in the Quickstarts repo.
git clone https://github.com/dapr/quickstarts.git
Step 2: Retrieve the secret
In a terminal window, navigate to the order-processor
directory.
cd secrets_management/csharp/sdk/order-processor
Install the dependencies:
dotnet restore
dotnet build
Run the order-processor
service alongside a Dapr sidecar.
dapr run --app-id order-processor --components-path ../../../components/ -- dotnet run
Behind the scenes
order-processor
service
Notice how the order-processor
service below points to:
- The
DAPR_SECRET_STORE
defined in thelocal-secret-store.yaml
component. - The secret defined in
secrets.json
.
// Program.cs
const string DAPR_SECRET_STORE = "localsecretstore";
const string SECRET_NAME = "secret";
var client = new DaprClientBuilder().Build();
var secret = await client.GetSecretAsync(DAPR_SECRET_STORE, SECRET_NAME);
var secretValue = string.Join(", ", secret);
Console.WriteLine($"Fetched Secret: {secretValue}");
local-secret-store.yaml
component
DAPR_SECRET_STORE
is defined in the local-secret-store.yaml
component file, located in secrets_management/components:
apiVersion: dapr.io/v1alpha1
kind: Component
metadata:
name: localsecretstore
namespace: default
spec:
type: secretstores.local.file
version: v1
metadata:
- name: secretsFile
value: secrets.json
- name: nestedSeparator
value: ":"
In the YAML file:
metadata/name
is how your application references the component (calledDAPR_SECRET_NAME
in the code sample).spec/metadata
defines the connection to the secret used by the component.
secrets.json
file
SECRET_NAME
is defined in the secrets.json
file, located in secrets_management/csharp/sdk/order-processor:
{
"secret": "YourPasskeyHere"
}
Step 3: View the order-processor outputs
As specified in the application code above, the order-processor
service retrieves the secret via the Dapr secret store and displays it in the console.
Order-processor output:
== APP == Fetched Secret: [secret, YourPasskeyHere]
Pre-requisites
For this example, you will need:
- Dapr CLI and initialized environment.
- Java JDK 11 (or greater):
- Oracle JDK, or
- OpenJDK
- Apache Maven, version 3.x.
Step 1: Set up the environment
Clone the sample provided in the Quickstarts repo.
git clone https://github.com/dapr/quickstarts.git
Step 2: Retrieve the secret
In a terminal window, navigate to the order-processor
directory.
cd secrets_management/java/sdk/order-processor
Install the dependencies:
mvn clean install
Run the order-processor
service alongside a Dapr sidecar.
dapr run --app-id order-processor --components-path ../../../components/ -- java -jar target/OrderProcessingService-0.0.1-SNAPSHOT.jar
Behind the scenes
order-processor
service
Notice how the order-processor
service below points to:
- The
DAPR_SECRET_STORE
defined in thelocal-secret-store.yaml
component. - The secret defined in
secrets.json
.
// OrderProcessingServiceApplication.java
private static final String SECRET_STORE_NAME = "localsecretstore";
// ...
Map<String, String> secret = client.getSecret(SECRET_STORE_NAME, "secret").block();
System.out.println("Fetched Secret: " + secret);
local-secret-store.yaml
component
DAPR_SECRET_STORE
is defined in the local-secret-store.yaml
component file, located in secrets_management/components:
apiVersion: dapr.io/v1alpha1
kind: Component
metadata:
name: localsecretstore
namespace: default
spec:
type: secretstores.local.file
version: v1
metadata:
- name: secretsFile
value: secrets.json
- name: nestedSeparator
value: ":"
In the YAML file:
metadata/name
is how your application references the component (calledDAPR_SECRET_NAME
in the code sample).spec/metadata
defines the connection to the secret used by the component.
secrets.json
file
SECRET_NAME
is defined in the secrets.json
file, located in secrets_management/python/sdk/order-processor:
{
"secret": "YourPasskeyHere"
}
Step 3: View the order-processor outputs
As specified in the application code above, the order-processor
service retrieves the secret via the Dapr secret store and displays it in the console.
Order-processor output:
== APP == Fetched Secret: {secret=YourPasskeyHere}
Pre-requisites
For this example, you will need:
Step 1: Set up the environment
Clone the sample provided in the Quickstarts repo.
git clone https://github.com/dapr/quickstarts.git
Step 2: Retrieve the secret
In a terminal window, navigate to the order-processor
directory.
cd secrets_management/go/sdk/order-processor
Install the dependencies:
go build .
Run the order-processor
service alongside a Dapr sidecar.
dapr run --app-id order-processor --components-path ../../../components/ -- go run .
Behind the scenes
order-processor
service
Notice how the order-processor
service below points to:
- The
DAPR_SECRET_STORE
defined in thelocal-secret-store.yaml
component. - The secret defined in
secrets.json
.
const DAPR_SECRET_STORE = "localsecretstore"
const SECRET_NAME = "secret"
// ...
secret, err := client.GetSecret(ctx, DAPR_SECRET_STORE, SECRET_NAME, nil)
if secret != nil {
fmt.Println("Fetched Secret: ", secret[SECRET_NAME])
}
local-secret-store.yaml
component
DAPR_SECRET_STORE
is defined in the local-secret-store.yaml
component file, located in secrets_management/components:
apiVersion: dapr.io/v1alpha1
kind: Component
metadata:
name: localsecretstore
namespace: default
spec:
type: secretstores.local.file
version: v1
metadata:
- name: secretsFile
value: secrets.json
- name: nestedSeparator
value: ":"
In the YAML file:
metadata/name
is how your application references the component (calledDAPR_SECRET_NAME
in the code sample).spec/metadata
defines the connection to the secret used by the component.
secrets.json
file
SECRET_NAME
is defined in the secrets.json
file, located in secrets_management/python/sdk/order-processor:
{
"secret": "YourPasskeyHere"
}
Step 3: View the order-processor outputs
As specified in the application code above, the order-processor
service retrieves the secret via the Dapr secret store and displays it in the console.
Order-processor output:
== APP == Fetched Secret: YourPasskeyHere
Tell us what you think!
We’re continuously working to improve our Quickstart examples and value your feedback. Did you find this Quickstart helpful? Do you have suggestions for improvement?
Join the discussion in our discord channel.
Next steps
- Use Dapr Secrets Management with HTTP instead of an SDK.
- Learn more about the Secrets Management building block
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.